Master View and Page View
You see how you can render any HTML en-block to the browser.
In a web application the pages usually contain common elements, that define the basic structure and the frame of every page.
These elements that frame each page can be abstracted out and re-used commonly among many pages.
This helps to maintain better resource economy and change management.
Please take a look at the sample's result now, and we will observe how it is assembled.
See the results in action by clicking here.
Cutting the HTML to Master and View parts
First we have to separate the common parts that is going to be our Master view and the content part that may be changing, this is going to be the page view.
Here are the two files:
The Master HTML only contains the , the head element, the navigation section, the footer and the script references.
- The opening and closing html tags
- The head Element and its content
- The Navigation section
- The Footer section
- The script references
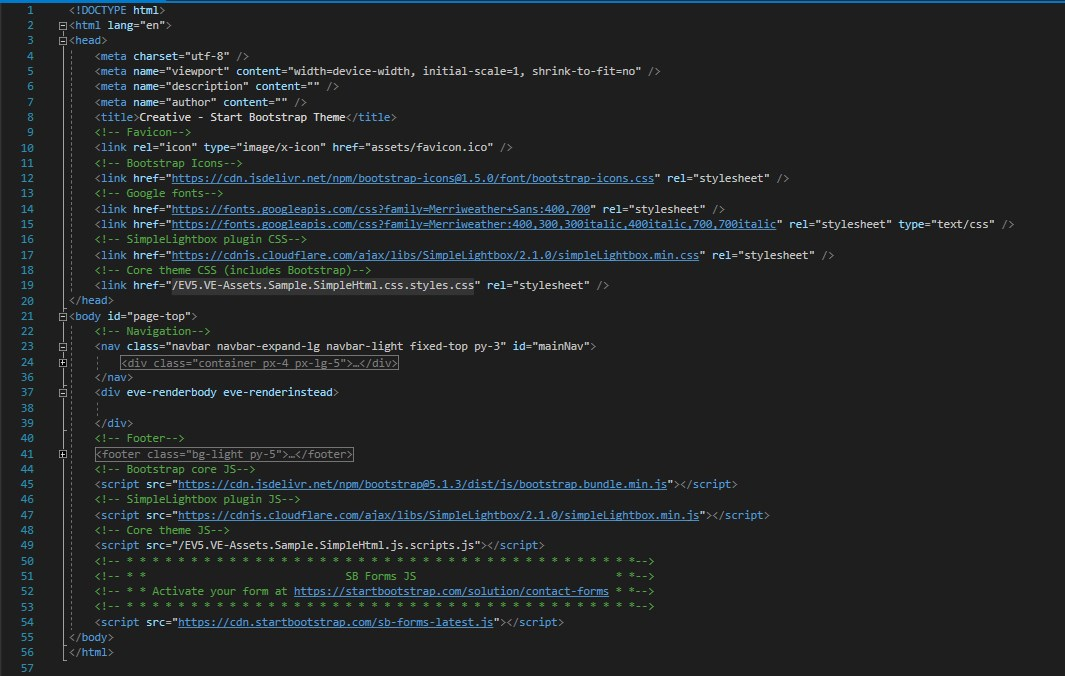
The element with the "eve-renderbody" attribute marks the place where the page content part should be placed.
<div eve-renderbody eve-renderinstead> </div>
The page part contains the rest of the content, the sections for:
- The header part
- The About Section
- The Services section
- The Portfolio section
- The Call To Action section
- The contact section
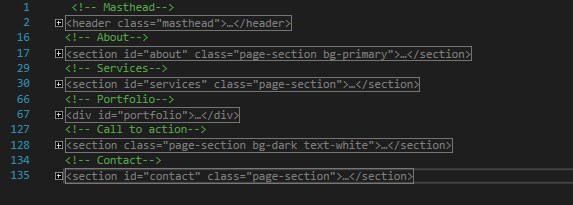
View Classes for Master View and Page View
Now that the markups are separated, let's create the view classes for both the master and page views.
First we have to establish which master page is used for this page. here is the code for the page view:
namespace EV5.Samples.ViewEngine.Views.SimpleMaster { [MasterView("eve-SimpleMaster.LandingPageMaster")] [MarkupName("EV5.VE-Assets.Sample.SimpleMaster.LandingPage.html")] [EmbeddedView("SimpleMaster.LandingPage")] public class LandingPageView : EmbeddedView { public override void ProcessView(ViewContext viewContext) { var node = this.HtmlDocument .Document .SelectSingleNode("//h1"); if (node != null) node.InnerHtml = "Changed in the Page view"; //*[@id="page-top"]/footer/div/div node = this.HtmlDocument .Document .SelectSingleNode(" //footer/div/div"); if (node != null) node.InnerHtml = "Changed in the Page view"; } } }The new element here is the "MasterView" Attribute that is denoting the master view to be used for this page.
The code for the master view is:
namespace EV5.Samples.ViewEngine.Views.SimpleMaster { [MarkupName("EV5.VE-Assets.Sample.SimpleMaster.LandingPageMaster.html")] [EmbeddedView("SimpleMaster.LandingPageMaster")] public class LandingPageMasterView : EmbeddedView { public override void ProcessView(ViewContext viewContext) { var node = this.HtmlDocument .Document .SelectSingleNode("//h1"); if (node != null) node.InnerHtml = "Changed in the Master view"; node = this.HtmlDocument .Document .SelectSingleNode("//*[@id=\"mainNav\"]/div/a"); if (node != null) node.InnerHtml = "Changed in the Master view"; } } }
Processing the Master View and Page View
In the above implementations you can see that the master view is trying to modify the navigation header and the Header Section text.
While the navigation header is part of the master markup, the Header section is not.
Also the page view is trying to modify the Header section, and the footer. Here the footer is not part of the page's markup.
If you click on the result you can see that the header section and the footer is successfully modified by the Page view.
While the master page could only modify the navigation header.
During the processing of the views the master page is always evaluated first, at that moment the Page content is not available yet.