Partial Views
To further abstract re-usability, you can use partial views. These are snippets of html, that can be inserted anywhere in master or page views.
You can also nest partial views into each other.
Cutting the HTML to partial views and View parts
In this example the landing pages uses the master page from the previous tutorial, but the page is assembled through partial views>
- Header partial view
- Content partial view
<!-- Masthead--> <div eve-partial="eve-EV5.VE-Assets.Sample.MasterAndPartials.Header.html" eve-renderinstead> </div> <!-- Content--> <div eve-partial="eve-EV5.VE-Assets.Sample.MasterAndPartials.Content.html" eve-renderinstead> </div>You can also see that here we are using the resource names of the views as this time there will be no code behind, we are just rendering the HTML as is, without further processing.
The content partial view contains
- About section partial view
- Services section partial view
- Html code for Portfolio, call to action and contact sections
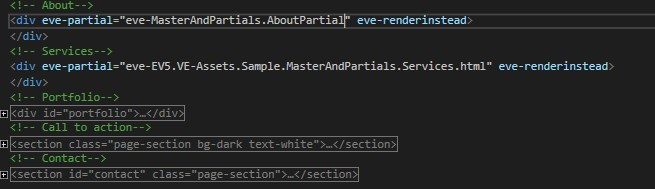
View Classes
We need a view class for the page view, because we have to set the master page to the one used in the previous example.
using EV5.Mvc; using EV5.Mvc.ViewEngine; using EV5.Mvc.MEF; using Microsoft.AspNetCore.Mvc.Rendering; namespace EV5.Samples.ViewEngine.Views.MasterAndPartial { [MasterView("eve-SimpleMaster.LandingPageMaster")] [MarkupName("EV5.VE-Assets.Sample.MasterAndPartials.LandingPage.html")] [EmbeddedView("MasterAndPartials.LandingPage")] public class LandingPageView : EmbeddedView { public override void ProcessView(ViewContext viewContext) { var node = this.HtmlDocument .Document .SelectSingleNode("//h1"); if (node != null) node.InnerHtml = "Changed in the Page view"; node = this.HtmlDocument .Document .SelectSingleNode(" //footer/div/div"); if (node != null) node.InnerHtml = "Changed in the Page view"; node = this.HtmlDocument .Document .SelectSingleNode("//*[@id=\"ctatext\"]"); if (node != null) node.InnerHtml = "Changed in the Page view"; } } }We will use the resource names for each partial view, thus there is no need for further view classes. Except the About section partial view. We will use this class to demonstrate the processing order of master, paga, and partial views.
using EV5.Mvc; using EV5.Mvc.ViewEngine; using EV5.Mvc.MEF; using Microsoft.AspNetCore.Mvc.Rendering; namespace EV5.Samples.ViewEngine.Views.MasterAndPartial { [MarkupName("EV5.VE-Assets.Sample.MasterAndPartials.About.html")] [EmbeddedView("MasterAndPartials.AboutPartial")] public class LAboutpartialView : EmbeddedView { public override void ProcessView(ViewContext viewContext) { var node = this.HtmlDocument .Document .SelectSingleNode("//h1"); if (node != null) node.InnerHtml = "Changed in the About Partial view"; //*[@id="page-top"]/footer/div/div node = this.HtmlDocument .Document .SelectSingleNode(" //footer/div/div"); if (node != null) node.InnerHtml = "Changed in the About Partial view"; } } }
Processing the Master View, Page View, and Partial views
In the above implementations you can see that the master view is trying to modify the navigation header and the Header Section text.
While the navigation header is part of the master markup, the Header section is not.
Also the page view is trying to modify the Header section, and the footer. Here the footer is part of the master page. Also, the page view is trying to modify the content of the Content partial view.
The About page view is trying to modify the header section again, and the first h2 it finds.
If you click on the result you can see that the header section and the footer is successfully modified by the Page view.
While the master page could only modify the navigation header. Also, the content is successfully modified by the page view, while the About partial view could only modify its own content.
The order of processing is the following:
- First always the master page is evaluated
- Then the partial views of the page view are evaluated top to bottom
- Further nested Partial views are processed, with the general rule that parents have access to heir children's html.
- Lastly the page view is processed.
- As a final and separate step, sections are processed, but this is the subject of the next tutorial.